실습은 tic-tac-toc이라는 js 프로젝트를 마이그레이션 하면서 typescript를 연습해본다.
raect+typescript는 이웅재님의 강의 참고하였습니다.
역시 이론과 실습이 결합할 때가 제일 재밌다!
처음 프로젝트를 생성할 때 부터 타입스크립 버전으로 셋팅하는것이 제일 깔끔하다.
1. create-react-app ts-test --scripts-version=react-scripts-ts
create-react-app + 프로젝트 이름 + --scripts-version=react-scripts-ts
추가
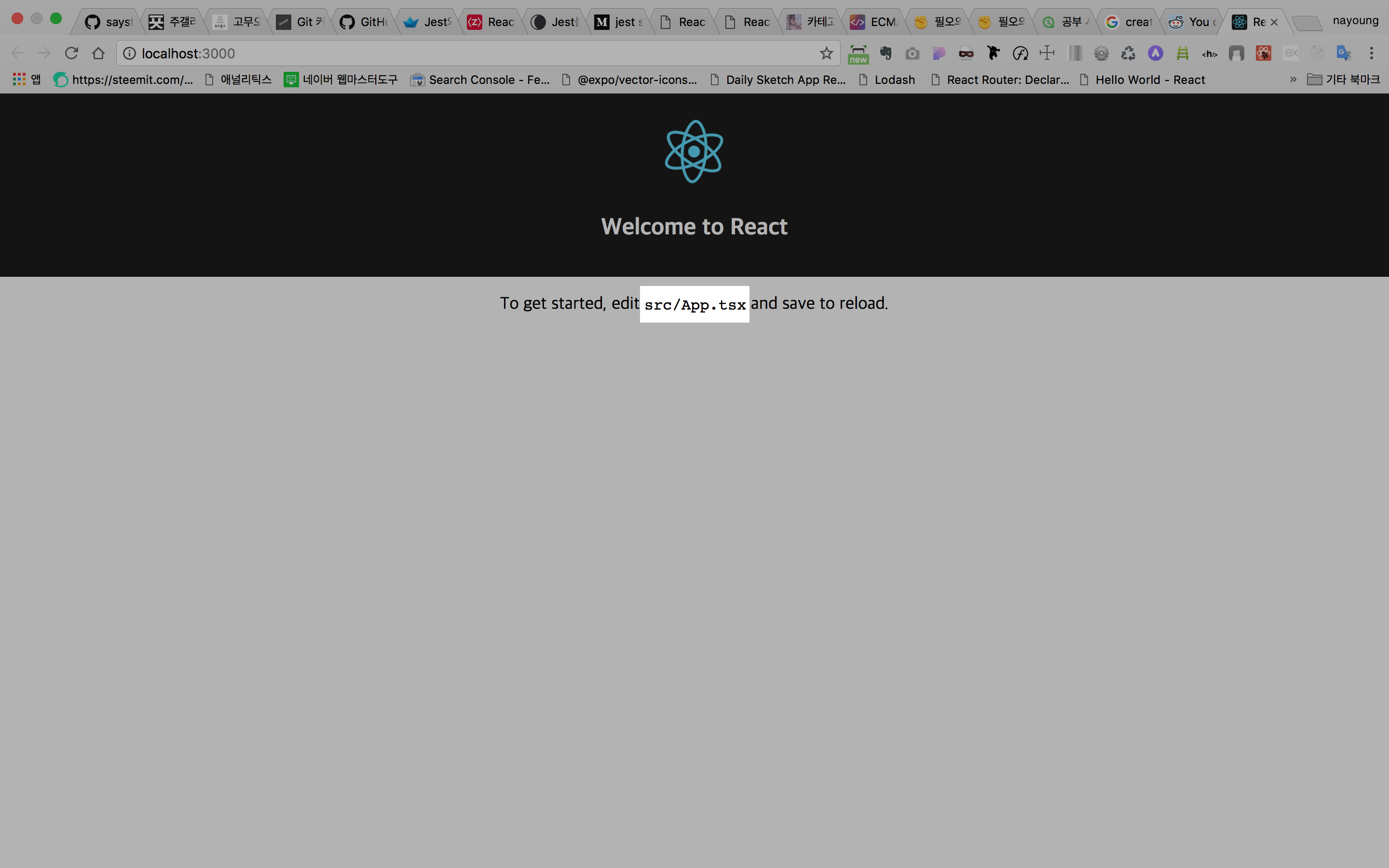
- react에서 사용하는 PropTypes를 사용하지 않아도 된다.
PropTypes
컴포넌트 에서 원하는 props 의 Type 과 전달 된 props 의 Type 이 일치하지 않을 때 콘솔에서 오류 메시지가 나타나게 하고 싶을 땐, 컴포넌트 클래스의 propTypes 객체를 설정하면 됩니다. 또한, 이를 통하여 필수 props 를 지정할 수 있습니다. 즉, props 를 지정하지 않으면 콘솔에 오류 메시지가 나타납니다.
1 | // Content.js |
2. state와 props는 interface로 처리를 해야한다.
class에서 입출력되는 타입을 검토하기 위해서
배열의 요소가 객체일 경우
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21interface GameState {
history: Array<{ squares: Array<string> }> // Array 요소가 객체일 경우 이렇게 타입을 지정해준다.
stepNumber: number
xIsNext: boolean
}
export default class Game extends React.Component<GameProps, GameState> {
constructor() {
super()
this.state = {
history: [
{
squares: Array(9).fill(null),
},
],
stepNumber: 0,
xIsNext: true,
}
}
...
}return에 대한 출력값을 타입으로 명시할때
JSX.Element
return 내부가 JSX문법이므로 JSX.Element로 명시하였다.
1 | export default class Game extends React.Component<GameProps, GameState> { |